Lecture 16
1.0 - Runtime Stack Organisation
- How do we generate code for procedure calls, their parameters and return values?
- The stack is used for evaluating expressions and storing their results, like we’ve seen in previous lectures.
- The stack is also used for storing activation records for procedure calls, and their corresponding return address (the code that we return to executing, after execution of the procedure call) as well as how we store local variables, and other variables.
1.1 - Stack Frame Organisation
- Note that in this example, we denote that the stack grows downwards (that is, the top of the stack is at the bottom of the page).
- At the top of the stack, we have a section that is currently being used for expression evaluation - this is part of the activation record for the procedure that is currently being executed.
- The purple section indicated is the stack frame of the procedure that is currently being executed.
- Above this is the stack frame for the procedure that called the procedure that is currently being executed.
- At the very bottom of the stack is the stack frame for the main method - the main method isn’t being called by any other method, so there aren’t any stack frames above it.
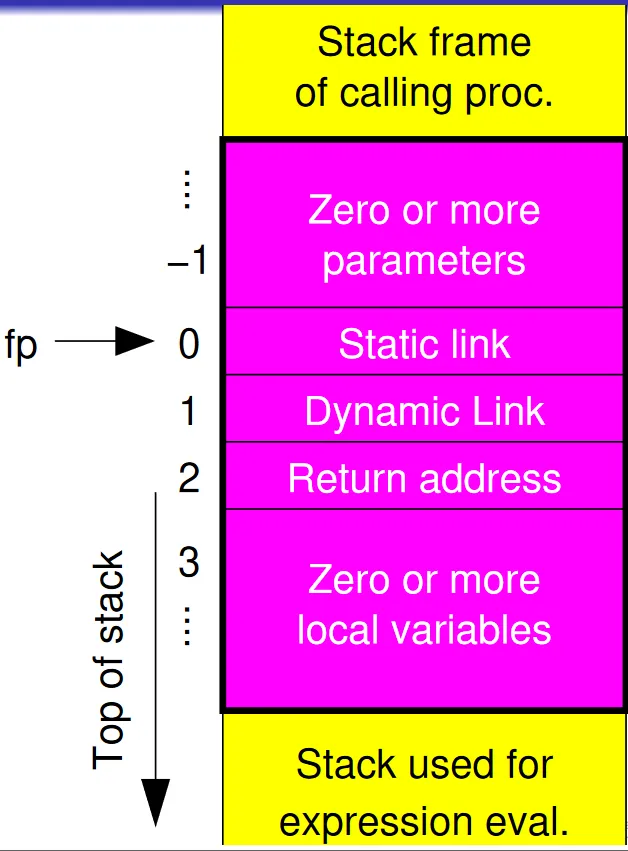
1.2 - Contents of Activation Record
Parameters
There is space at the top of the stack frame to store the value of parameters that have been passed into the procedure.- We conventionally denote that our procedure parameters are stored at a negative offset from the frame pointer.
- These parameters are stored in reverse order of their occurrence (i.e. the first parameter stored at
) - If the first parameter has a size of 1 word, then the second parameter would be stored at
- If the first parameter has a size of 1 word, then the second parameter would be stored at
- The frame pointer of a procedure points to the static link - we use this to access other stack frames in order to access variables that are not local to the current procedure.
Static Link
Used to access other stack frames to access variables that are not local to the current procedure.- The frame pointer points to the static link (i.e. the static link is stored at an offset of 0 from the frame pointer).
Dynamic Link
Link to the activation record of the procedure that called this current procedure.- We use the Dynamic Link to restore the frame pointer to the previous procedure (after execution of the current procedure is completed)
Return Address
Remember the address of the code that came after this procedure call. That is, the Return Address gives us the program counter position to return to after executing the procedure call.Local Variables
We allocate space for the local variables defined in a procedure.- Expression Evaluation The section mentioned before, that
1.3 - Calling a Procedure
- The
parameters
to the procedure are pushed onto the stack by the calling procedure.- Must push the parameters onto the stack in the reverse order, to preserve the convention that the first parameter is stored at an offset of -1 from the frame pointer.
- The
static link
(of the procedure being called) is pushed onto the stack - The current
frame pointer
is pushed onto the stack to create thedynamic link
- Set the
frame pointer
of the stack machine is set so that it contains the address of the start of the new stack frames (that is, we want the new frame pointer to point to the static link). - The current value of the program counter (which is the address of the instruction after the call) is pushed onto the stack to form the
return address
. - The
program counter
is set to the address of the procedure- From this point onward, when we execute code (stack machine instructions), we ??
- Space is allocated on the stack for any local variables, using the
ALLOC_STACK
instruction- The procedure (that is being called) sets up the space on the stack for the local variables.
- The CALL instruction implements steps 3 to 6 of the above sequence.
1.4 - Returning from a Procedure
- The program counter is set to the return address in the current activation record
- Restore the program counter to the return address of the current activation record.
- The frame pointer is set to the dynamic link
- The position on the stack of the activation record of the calling procedure
- The stack pointer is set so that all of the space used by the stack frame (but not the parameters) is popped from the stack (essentially, deallocate the space from the stack).
- Execution continues at the instruction addressed by the restored program counter
- After return, the calling procedure handles deallocating any parameters (using the instruction DEALLOC_STACK).
- The RETURN instruction handles steps 1 - 4 above. Note that there is no need to explicitly deallocate the variables as setting the stack pointer (in Step 3) achieves this.
1.5 - Procedure Call Example
-
Consider the following PL0 program - let’s run through the procedure call and return steps described above.
var n: int; f: int; procedure fact() = begin if n = 0 then f := 1 // 0! = 1 else begin // Calculate (n - 1)! n := n - 1; call fact(); n := n + 1; // restore n // calculate n! f := f * n end; end; // fact begin // Main n := 2; call fact(); write f end
-
Note that this example doesn’t have any parameters or return variables.
-
Since we don’t define any local variables, we use the global variables
n
andf
.- We use n as a sort of parameter; it is defined in the parent scope
- We use f as a sort of return value; it is defined in the parent scope
1.5.1 - Begin Executing the Main Method
begin // Main
n := 2;
call fact();
write f
end
- Set up an activation record for the main method.
- The static link, dynamic link and return address don’t have a value for the main method - the main method is not called by a procedure.
- We allocate space on the stack for the local variables (n and f)
- The variable
is stored at an offset of (address ) - The variable
is stored at an offset of (address of )
- The variable
- We then execute the code
which assigns the variable n the value of 2 - this is trivial as it is defined as a local variable, and therefore, is stored at some offset from the current frame pointer.
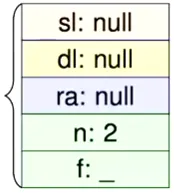
- Procedure call to fact()
1.5.2 - Set Up Procedure Call to Fact
- To set up the activation record for the procedure fact(), we need to do a few things:
Static Link
Address on the stack of the activation record for the most recent invocation of the enclosing procedure (in this case, Main). Therefore, in this case, we point to the start of the Main procedure’s activation record. Used to access variables in the parent scope(s).Dynamic Link
Address of activation record of the calling procedure.Return Address
Should be in between the call fact(); and write f statements. We refer to this position as “Main”
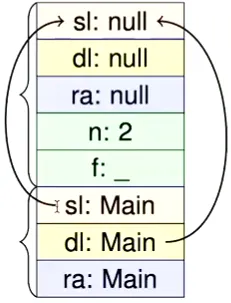
1.5.3 - Executing Procedure Fact
-
The first statement to execute in the procedure fact is the check
in which we need to access the variable . if n = 0 then
-
Since it’s not defined in the current scope (it’s defined in the parent scope), we use the static link to access the variable.
-
Since it is defined at one static level above the current procedure, we follow the static link pointer of the current activation record, to get the position on the stack of where the frame is stored that contains
-
We access
using the above stack pointer lookup (and knowing that it’s stored at an offset of +3) -
Since its value is not equal to zero, we continue execution by executing the code in the “else” body.
Else Body Execution
-
In this block of code, the first statement that we want to evaluate requires obtaining the value of the variable n.
n := n - 1;
-
Once again, as it is not defined in the current scope, we repeat the steps shown above to access its value.
1.5.4 - Second Call to Fact()
- As in the set-up call section before, we need to set up another activation record for the new (recursive) call to the fact() procedure.
- Note here that the static link and dynamic link of both fact() activation records are different.
- The return address “fact” refers to a position in between
call fact();
andn := n + 1;
- Note here that if we had a local variable “n” inside fact, that would overshadow the variable “n” defined in Main.
- Additionally Main is at static level 1, and fact1 and fact2 at static level 2.
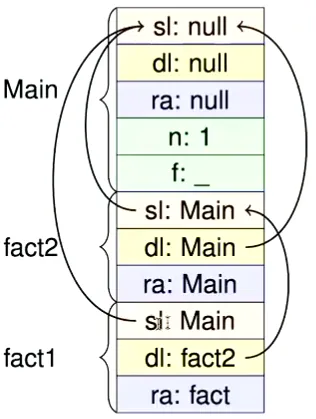
1.5.5 - Executing Fact Procedure for the Second Time
- We follow the static links back to Main, to find the value of the variable
. - We find that it has a value of 1, and therefore execute the “Else” block once again.
- We call fact() again, which requires the set-up of another activation record.
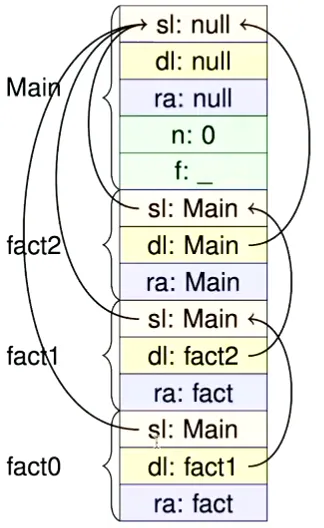
-
Observe that in this new activation record, the static link is the same, the Dynamic link is to the previous invocation and the return address is the point after the procedure call to fact() in fact1.
-
In executing the code, we set the value of the variable f to 1 (this is effectively our return value in this example).
f := f * n
-
In returning to the previous method, we need to restore the program counter and frame pointer (using the dynamic link).
-
We remove the activation record from the stack, and continue the execution of the code ...
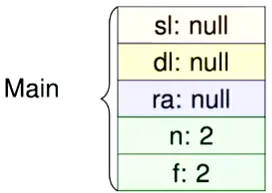
1.6 - Generating Code for Non-Local Variable Access
// Push the static link for the current frame
// (i.e. the address of frame for procedure C)
ZERO
LOAD_FRAME
// Replace with static link of next outer frame
// i.e. the address of the frame for the main program
LOAD_ABS
// Add offset of x
LOAD_CON 3
ADD
// Convert from an offset from fp
TO_LOCAL
// Load the value of x
LOAD_FRAME
- Consider the following PL0 program, in which we are in the procedure “fact” and would like to access the variable “x” defined in the scope of the main method.
- We observe that the procedure fact() is at static level 3:
- The main method is at Static Level 1
- The procedure C is at Static Level 2
- The procedure fact is at SL 3.
- Additionally, we observe that the variable “x” is defined at static level 1.
- Therefore, we need to follow the static links back two times in order to get the address of the activation record in which “x” is stored.
- Finally, we know that the variable “x” is stored at a known offset from this position.
var x: int; // first argument to C
y: int; // second argument to C
res: int; // result from C
procedure C() =
var n: int; // argument to fact
f: int; // result from fact
procedure fact() =
begin
// assume 0 <= n
if n = 0 then
f := 1
// f = n!
else // 0 < n
begin
n := n - 1;
call fact();
// f = n!
n:= n + 1;
f := f * n
// f = n!
end
end; // fact
begin // C
n := x;
call fact();
// f = x!
res := f;
n := y;
call fact();
// f = y!
res := res / f;
// res = x! / y!
n := x - y;
call fact();
// f = (x-y)!
res := res / f
// res = x! / (y! * (x-y!))
end; // C
begin // main program
x := 6;
y := 2;
call C();
write res
end
1.6.1 - visitVariableNode method
-
To generate code for a variable reference, we essentially need to push the address of the variable as an offset from the current frame pointer onto the stack.
/** * Generate code for a variable reference. * It pushes the address of the variable as an offset from the frame pointer */ public Code visitVariableNode(ExpNode.VariableNode node) { beginGen("Variable"); SymEntry.VarEntry var = node.getVariable(); Code code = new Code(); code.genMemRef(staticLevel - var.getLevel(), var.getOffset()); endGen("Variable"); return code; }
-
The mechanics of pushing this value onto the stack is mainly handled by the genMemRef method.
public void genMemRef(int levelDiff, int offset) { if (levelDiff == 0) { /* A local variable, so just load the offset from the frame pointer */ genLoadConstant(offset); } else { /* Generate code to load the address of the frame containing the variable. */ loadFrameAddress(levelDiff); /* Add the offset of the variable to get absolute address of variable. */ genLoadConstant(offset); generateOp(Operation.ADD); /* Convert from absolute address to an address relative to the * current frame pointer. */ generateOp(Operation.TO_LOCAL); } }
- In this method, we need to handle two discrete cases - where the variable is a local variable, and when it is not.
Variable is Local
If the variable is local, then. - In this case, we load the variable’s offset from the start of it’s defined scope.
Variable is not Local
If the variable is not local, then we:- Use the
loadFrameAddress(int levelDiff)
method to obtain the memory address of the scope in which the variable is defined. - Add the variable’s offset to this memory address (observe that this is an absolute memory address).
- We then use the
Operation.TO_LOCAL
operation to convert the absolute memory address to a local memory address (that is, an offset from the current frame pointer).
- Use the
-
We know that our stack machine code for accessing a non-local variable x (that is two static levels above the current activation record) is given as:
// Push the static link for the current frame // (i.e. the address of frame for procedure C) ZERO LOAD_FRAME // Replace with static link of next outer frame // i.e. the address of the frame for the main program LOAD_ABS // Add offset of x LOAD_CON 3 ADD // Convert from an offset from fp TO_LOCAL // Load the value of x LOAD_FRAME
- We know that the static link of the current activation record is stored at an offset of 0 of the current frame pointer. Therefore, using the
ZERO
andLOAD_FRAME
instructions, we essentially push the static link of the current frame onto the stack. This address is the start of the activation record that is one static level above the current activation record. - We use the
LOAD_ABS
instruction to load the static link of this new activation record onto the top of our stack. - At this point, the value on top of the stack is the address of the (start of the) new activation record in which the variable “x” is defined. We use the
LOAD_CON 3
andADD
instructions to add the offset of x to the address stored on the top of the stack. - We then convert the absolute memory address to an offset from the current frame pointer using the
TO_LOCAL
instruction. - We then use the
LOAD_FRAME
command to load the value stored at this new memory address.
- We know that the static link of the current activation record is stored at an offset of 0 of the current frame pointer. Therefore, using the
-
LoadFrameAddress
method (i.e. an implementation of above)./** * Generate the code to chase the static link chain. * * @param levelDiff the number of frames to chase back. * requires 0 <= levelDiff */ public void loadFrameAddress(int levelDiff) { assert 0 <= levelDiff; if (levelDiff == 0) { /* The static link is the current frame pointer */ generateOp(Operation.ZERO); generateOp(Operation.TO_GLOBAL); } else { /* Load the static link of the current frame */ generateOp(Operation.ZERO); generateOp(Operation.LOAD_FRAME); /* Follow the static link chain back levelDiff-1 times */ for (int i = levelDiff - 1; i > 0; i--) { generateOp(Operation.LOAD_ABS); } } }
- In the case where we want to load the frame address of the current frame, we use the
TO_GLOBAL
method with an offset of 0. - In the case where we want to load the frame address of a frame that is up multiple static levels, we use the
LOAD_FRAME
instruction to push the value of the static link onto the stack.- We then append a new
LOAD_ABS
instruction for each static level we want to traverse. - This essentially goes down a static link every time a
LOAD_ABS
instruction is called, as the value stored at a static link is the address of the next static link (start of the frame).
- We then append a new
- In the case where we want to load the frame address of the current frame, we use the