Lecture 17
1.0 - Procedure Calls and Returns for Stack Machine
-
Consider the case where we make a call to a procedure that has both parameters and a return value.
procedure fact(n : int) : int = var f : int; begin if n = 0 then f := 1 // 0! = 1 else f : fact(n-1)*n; end; //fact begin // Main write fact(2) end
-
In running this code, we initially set up an activation record containing the static link, dynamic link and return address of the main method.
-
If the Main method had local variables, they would be allocated a space under the return address.
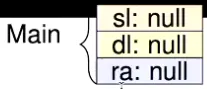
- In executing the main method, we need to evaluate the expression
write fact(2)
. - In order to do this, we make a procedure call to the procedure fact, with a parameter of 2.
- Before constructing the activation record, we need to allocate a space on the stack for the return value (here, denoted as “_”) as well as for the parameter n (we also push the value of the parameter onto the stack).
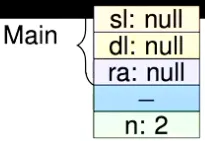
- We then call the procedure fact, setting up its activation record.
Static Link
Pointer to the most recent invocation of the enclosing procedure.Dynamic Link
Pointer to the calling procedure.Return Address
The point in the program to return to after execution of procedure fact. Here, we abbreviate the point afterwrite fact(2)
asMain
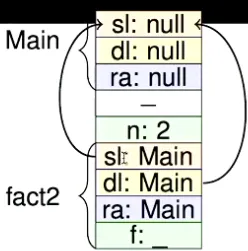
- In executing the procedure fact, we first want to test if
is true or false. Since is the first parameter passed to the method, we know that it is stored at a location of . - Since
, we know that we need to execute the “else” branch, which invokes the procedure fact once again.
- In setting up the second invocation of the procedure fact, we need to:
- Allocate space for the return value (denoted here as “_”)
- Allocate space for the parameter (denoted here as n: 1)
- After allocating the aforementioned space, we create the activation record for the new invocation.
- Note here that the parameter to this invocation of fact is different to the previous.
Static Link
Pointer to the most recent invocation of the enclosing procedure - this is the procedure Main in this case.Dynamic Link
Pointer to the calling procedure - in this case, the previous invocation of the procedure fact called this procedure.Return Address
The point in the program to return to after the exec. In this case, the point “fact” refers to the point after the call to fact, but before the evaluation of the expressionfact(...) * n
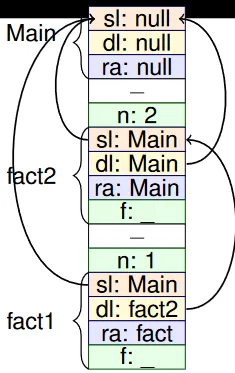
- In executing the procedure fact, we first want to test if
is true or false. As before, since is the first parameter passed to the method, we know that it is stored at a location of - Since
, we know that we once again need to execute the “else” branch, which invokes the procedure fact once again.
- In setting up the third invocation of the procedure fact, we once again allocate space for the return value and return parameter.
- After allocating the aforementioned space, we create the activation record for the new invocation.
Static Link
Pointer to the most recent invocation of the enclosing procedure - this is the procedure Main in this case.Dynamic Link
Pointer to the calling procedure - in this case, the previous invocation of the procedure fact called this procedure.Return Address
The point in the program to return to after the exec. In this case, the point “fact” refers to the point after the call to fact, but before the evaluation of the expressionfact(...) * n
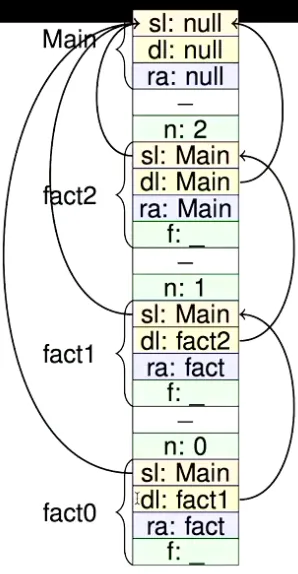
- In executing the procedure fact, we first want to test if
is true or false. As before, since is the first parameter passed to the method, we know that it is stored at a location of - Since
, we know that we need to execute the “if” branch, which sets the value of the local variable to 1. - We then execute the return statement;
return f
. To achieve this, we write the value 1 onto the stack reserved for the return value in the spaced allocated by the invoking procedure.
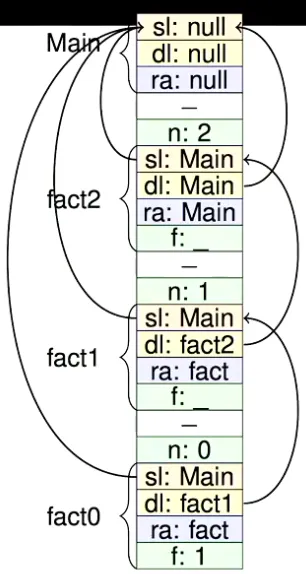
- We then execute the required behaviours when returning from a procedure:
- Restore the program counter using the return address.
- We restore the frame pointer using the dynamic link
- We pull the completed procedure’s activation record off the stack.
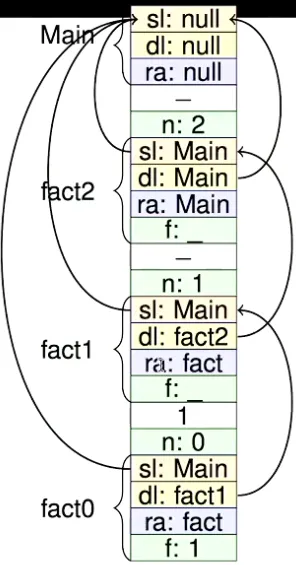
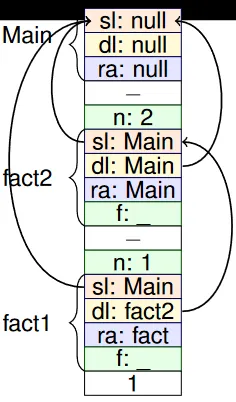
-
Observe that after these steps have been completed (figure on the right), the return value is on top of the stack.
-
We can now use the stack to evaluate the value of
and assign that to the local variable . -
This value is then recursively returned (for the remaining two invocations of the procedure fact):
- In returning this value, we write this to the return value space in the position
so that it is available to the calling procedure. - Once again, we restore the program counter (using the return address), frame pointer (using the dynamic link) and pull the completed procedure’s activation record off the stack.
- In returning this value, we write this to the return value space in the position
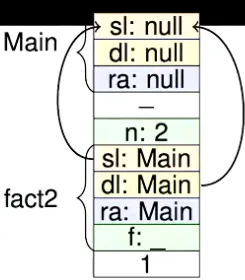
- We once again evaluate the value of
and return that value - Once again, we restore the program counter (using the return address), frame pointer (using the dynamic link) and pull the completed procedure’s activation record off the stack.
- We have returned to the main method, with the value of fact(2) on the stack. We can then write out the value, and return out of the main method!
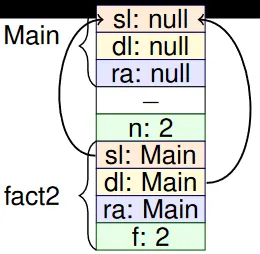
2.0 - Procedure Call Parameters
2.1 - Parameter Passing Mechanisms
Formal Parameters
The parameters used in the declaration of the procedure in its header.- The formal parameter names and types are used to provide access to the parameters from within the body of a procedure
Actual Parameters
The parameters actually passed to a procedure on a call. These may differ from call to call.
2.2 - Implementation of Parameters - Call-by-Value Parameters
-
For call-by-value parameters, the actual parameters are expressions that are evaluated (and if necessary coerced to the type of the corresponding formal parameter)
-
The values of the actual parameters are loaded onto the stack as part of the calling sequence, before the new stack frame is set up.
-
Once the procedure is called and the stack frame has been established the formal parameters of the procedure can be accessed like local variables, except that they usually have negative offsets from the frame pointer.
-
Accesses to the formal parameter access the location on the stack containing the value of the corresponding actual parameter.
-
Consider the following PL0 code:
procedure fact(n : int) : int = begin if n = 0 then return 1 else return n * fact(n-1) end; begin // Main program write fact(2) end
- Formal parameter n of type integer
- Return value of type integer
- When we call fact(2), we pass it the actual parameter, which is an expression (in this case, the expression evaluates to 2).
- This value (2) becomes the value of the formal parameter n in the procedure fact for this particular invocation
- When we call fact(n-1) for the first time (when n = 2), the actual parameter is the expression (n-1) which evaluates to 1.
- Therefore, the value of the formal parameter for this invocation is 1.
2.2 - Function Results
-
A function returns a result, and hence can be used as part of an expression.
n * fact(n-1)
-
Hence, the result of a function call should be left on top of the stack without any other information to do with the call being left underneath.
-
For this reason, space is allocated for a function result on the top of the stack before parameters are loaded onto the stack and the stack frame is set up.
-
Code generation for call/return in PL0 is specified in the stack machine handout.
2.3 - Types of Parameter Passing Mechanisms
Call by Const
The formal parameter acts as a read-only local variable that is initially assigned the value of the actual parameter expression. This is the same as Call by Value, except you are not able to modify the value of the parameter in the procedure,Call by Value
The formal parameter acts as a local variable that is initially assigned the value of the actual parameter expression. Assigning to the formal parameter does not change the actual parameter.Call by Result
The formal parameter acts as a local variable whose final value is assigned to the actual parameter variable. That is, we write the value that we calculate for the parameter to the actual parameter.Call by Value-Result
A single parameter acts as both a value and a result parameter. When the procedure is called, the formal parameter acts as a local variable that is initially assigned the value of the actual parameter expression. However, we assign the value of the parameter to the variable that we were passed.Call by Reference
The formal parameter is really the address of the actual parameter variable; all references to the formal parameter are applied (via that address) to the actual parameter variable immediately. (In Pascal, this is known as a var parameter) as inC
Call by Sharing
is the same as call-by-value but what is passed is a reference to an object (as in Java) rather than the value of the (attributes) of the object. This is commonly known as “Call by Reference” as inJava
.Call by Name
The actual parameter expression is evaluated every time the formal parameter is accessed. That is, we pass in an expression and evaluate it every time.Passing Procedures (or Functions) as Parameters
pass the address of the procedure as well as the static link for the procedure’s environment, i.e. the static link to be used when calling the procedure.- When we pass a procedure in a procedural language like PL0, we also need to have access to variables defined in nested scopes - this is why we need to pass the static link.
Returning Procedures (or Functions)
Return the address of the procedure as well as the static link for the procedure’s environment, i.e. the static link to be used when calling the procedure; note that this requires the environment of the returned procedure to be maintained, which means that the simple stack-based allocation of frames that is currently implemented is not sufficient to implement returning procedures.
2.4 - Java Mechanics
2.4.1 - Pass by Value
- Note that in the code snippet below, we get that the value of x is 0 - that is, the
p_val
method doesn’t modify the actual variable.
static void p_val(int i) {
i = i++
}
public static void main(String[] args) {
int x = 0;
p_val(x);
System.out.println("value parameter x " + x);
}
2.4.2 - Pass by Sharing
- Java uses Pass by Sharing when the parameter is an object.
- That is, when the parameter is an object, Java passes the object as a reference.
- Therefore, when we update the internal state of the object, the value of that variable in all scopes is changed.
- However, this could lead to “Pointer Aliasing” which can cause bugs in code.
static class Int {
int val;
Int(int i) { val = i; }
}
static void p_ref(Int i) {
i.val = i.val + 1;
}
public static void main(String[] args) {
Int y = new Int(0);
p_ref(y);
System.out.println("reference parameter y " + y.val);
}
2.4.2 - Call by Sharing Issue - Pointer / Global Variable Aliasing.
-
Parameter Aliasing In languages (like Java) with call-by-sharing, the same reference to an object can be passed to two different parameters leading to one having two aliases for the same reference, often called pointer aliasing.
-
Global Variable Aliasing If a reference that is passed as a parameter to a procedure is also directly accessible as a global variable from the procedure. This leads to the procedure this leads to the procedure having two aliases for the one reference, i.e. pointer aliasing
-
The following demonstrates a case where parameter aliasing doesn’t occur - that is, the two parameters that we pass into the method are distinct.
static class Int { int val; Int(int i) { val = i; } } static void p_ref_ref(Int i, Int j) { i.val = 1; j.val = 2; } public static void main(String[] args) { Int y = new Int(0); Int z = new Int(0); p_ref_ref(y, z); System.out.println("Distinct reference parameters " + y.val + " " + z.val); // Distinct reference parameters 1 2 }
-
However, when we execute a case whereby the two values are the same, parameter aliasing occurs, where we update both variables:
- That is, in
i.val = 1
we set both the first and second formal parameter’s values to 1. - Additionally in
j.val = 2
we set both the first and second formal parameter’s values to 2.
static class Int { int val; Int(int i) { val = i; } } static void p_ref_ref(Int i, Int j) { i.val = 1; j.val = 2; } public static void main(String[] args) { Int y = new Int(0); p_ref_ref(y, y); System.out.println("Same reference parameter " + y.val); // Same reference parameter 2 }
- That is, in
-
Additionally, we could have global variable aliasing:
-
The following demonstrates a case where the parameter aliasing doesn’t occur (as the global variable and the parameter refer to different objects).
static Int global = new Int(0); static class Int { int val; Int(int i) { val = i; } } static void p_ref_global(Int i) { i.val = 1; global.val = 2; } public static void main(String[] args) { p_ref_global(y); System.out,.println("Distinct reference parameter and global " + y.val + " " + global.val); }
-
As opposed to the previous example, if we pass a global variable into a method that modifies the global variable and the parameter, we modify the variable twice, potentially over-writing our changes.
Suppose we have a class “Matrix” representing matrices.
Matrix Z = new Matrix(2,2); // rows, cols
Matrix X = new Matrix(new int[][]{{-2,-1},{1,2}}); // 2 rows, 2 cols
add(X, X, Z); // Aliasing present, but okay as we take the values from Matrix X and
// update matrix Z based on these values.
add(X, X, X); // Aliasing present, but behaviour is NOT as expected, as we're
// overwriting the values in a way that doesn't affect the end result.
// At the point where we over-write the values, we have already evaluated
// the value of what to write.
multiply(X, X, Z); // Aliasing present, but okay as we take the values from Matrix X and
// update matrix Z based on these values.
multiply(X, X, X); // Aliasing present, behaviour is NOT as expected as we're
// overwriting values used for the values of other cells -
// this changes the output.
2.4.3 - Call by Reference Issue - Variable Aliasing
Parameter Aliasing
in languages with call-by-reference - the same variable can be passed to two (or more) different parameters, leading to variable aliasing - two different formal parameter names referring to the same actual parameter variable. This leads to variable aliasing within the procedure itself.Global Variable Aliasing
If a variable is passed as the reference parameter to a procedure that can access the same variable as a global variable, then within the procedure there are two aliases for the same variable.