1.0 - Recurrences
1.1 - Divide and Conquer Algorithms
1.1.1 - Merge Sort
merge_sort(A, p, r)
sorts the sub-arraywhich uses the merge(A, p, q, r)
subroutinemerge(A, p, q, r)
takes the sorted sub-arraysand and merges them to produce the sorted array . - This assumes
merge(A, p, q, r)
iswhere - i.e. we’re measuring the input in terms of the number of items that need to be merged
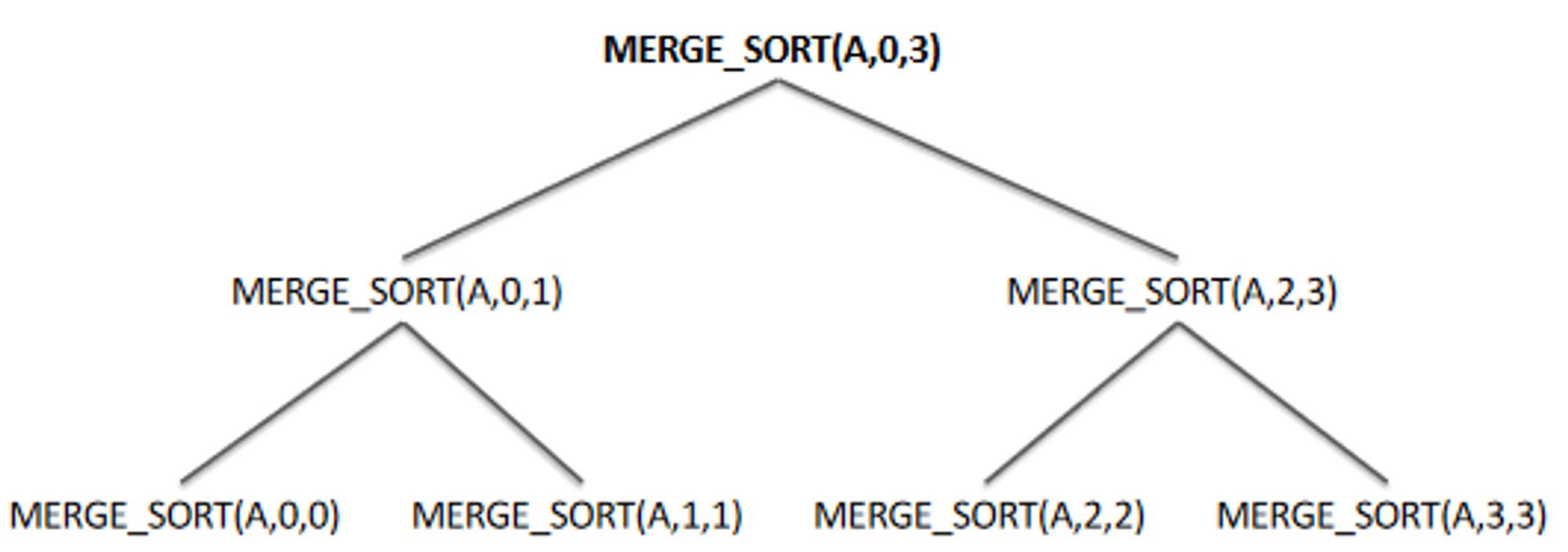
if p < r // If there are two or more elements to merge, we divide:
q = ⌊(p + r) / 2⌋ // Divide
merge_sort(A, p, q) // solve sub-problem recursively T(n/2) work
merge_sort(A, q+1, r) // solve sub-problem recursively T(n/2) work
merge(A, p, q, r) // combine
-
Let
-
If we have hit the base case, (we assume) that the method is going to take a constant amount of time to execute an return otherwise.
-
Otherwise, we have to perform
work to sort the two sub-problems, as well as the work required to merge the two sub-problems together. -
is shorthand for for some function
1.2 - Recurrences
-
To be well-defined, a recurrence needs:
- base case, and
- recursive case(s) that converge on the base case
-
Running time is usually bounded by a constant for constant-sized inputs, and so we often omit the base case, and assume that
where
is a constant.
1.1 - Divide and Conquer Algorithms
- Given a divide and conquer algorithm that:
- Takes
time to divide the problem, and - Takes a problem of size
, and - Breaks it into
parts, each of size - Takes
time to combine solutions to sub problems, we get the following recurrence:
- Takes
1.1.1 - Merge Sort
merge_sort(A, p, r)
sorts the sub-arraymerge(A, p, q, r)
takes the sorted sub-arraysand and merges them to produce the sorted array . - This assumes
merge(A, p, q, r)
iswhere
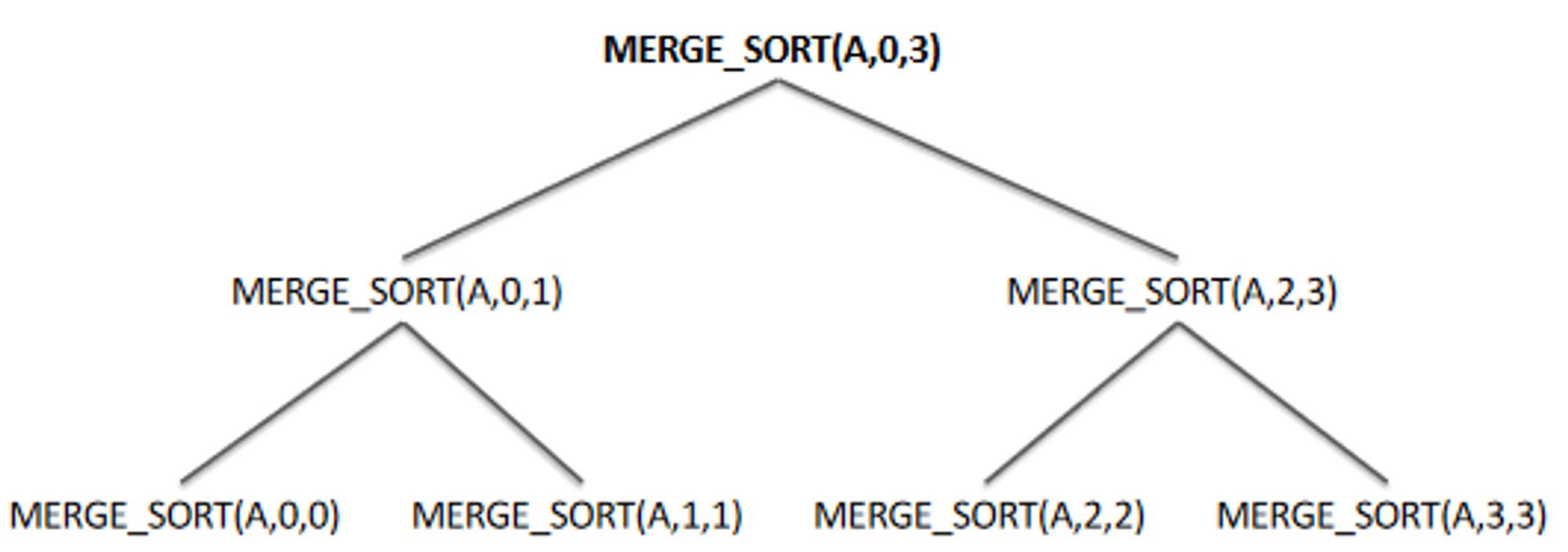
if p < r
q = ⌊(p + r) / 2⌋ // Divide
merge_sort(A, p, q) // solve sub-problem recursively
merge_sort(A, q+1, r) // solve sub-problem recursively.
merge(A, p, q, r) // combine
-
Let
-
is shorthand for for some function - That is,
is a yet-unknown function that is
- That is,