1.0 - Dynamic All-Pairs Shortest Paths Algorithms
- DIjkstra’s algorithm finds all shortest paths from one node
- The fastest implementation is
- Running Dijkstra’s Algorithm from all nodes is therefore
, however this cannot handle negative-weight edges. - Bellman-Ford is
so with an extra loop for each nodes gives - We can get
and handle negative-weight edges for all-pairs
1.1 - Recursive Definition of All-Pairs
-
To solve this problem, we first define some notation
- There are
vertices, with IDs - Uses an adjacency-matrix representation, with
as the weight of the edge from vertex to vertex - If no edge exists, then the weight is
, hence return as the weight of the shortest path if one does not exist
- There are
-
A path from vertex
to can be either: -
A path with no edges of weight
, e.g. for -
A path with no more than one edge consisting of:
- A path
, from vertex to some vertex , and - The edge
The weight of such path is
- A path
-
-
If the shortest path
from to has edges, then - The path from
to must have (at most) edges, and - It must be the shortest path from
to
- The path from
-
Let
repeat the weight of the shortest path from to with at most edges And hence,
-
Since each sub-problem is described by 3 parameters, we require a 3d matrix to store the solutions
1.1.1 - Naive, Slow Solution
-
We store
at in an array -
Calculate
, then from , then from etc slow_apsp(n) // L[m, i, j] is weight of the shortest path from i to j of at most m edges L = new int[n-1][n][n] // Initialise all elements to infinity. L[1] = weights for m = 2 to n - 1 d = L[m-1] d' = L[m] for i = 1 to n for j = 1 to n for k = 1 to n d'[i, j] = min(d'[i, j], d[i, k] + weight(k, j))
-
This algorithm is
which is the same as running the Bellman ford algorithm times.
1.1.2 - Improved Solution
- We calculate
then , using - Then
from - Then
from
faster_apsp(n):
// L[m, i, j] is the weight of the shortest path from i to j of at most m edges
L = new int[2(n-1) - 1][n][n]
L[1] = weights
m = 1
while m < n - 1
d = L[m]
d' = L[2m]
for i = 1 to n
for j = 1 to n
for k = 1 to n
d'[i, j] = min(d'[i, j], d[i, k] + d[k, j])
m = 2m
- Thus, this algorithm runs in
time
1.2 - Floyd Warshall Algorithm
-
Why did we define our sub-problem in terms of path length?
-
Instead, we can phrase our sub-problem in terms of which intermediate nodes are in the path
-
Let
be the shortest path from to , using only intermediate vertices - Remember that our vertices are conveniently ordered indices
- Remember that our vertices are conveniently ordered indices
-
We incrementally add a new node to the intermediate set.
-
Extending
to requires checking whether the path formed by: - Going from
and is better than already found.
- Going from
-
Let
be the weight of the shortest path from to , using only intermediate vertices $\small\text{shortestPath}(i, j, k)=\text{weight}(i, j)\ \text{shortestPath}(i, j, k+1)=\min\begin{cases}\text{shortestPath}(i, j, k)\ \text{shortestPath}(i, k+1, k) + \text{shortestPath}(k+1, j, k)\end{cases}$
$\def\t{\ \ \ \ } 1\ \text{floyd-warshall}(W)\ 2\ \t n = W.\text{rows}\ 3\ \t D^{(0)} = W\ 4\ \t \bold{for\ } k = 1 \bold{\ to\ } n\ 5\ \t\t \text{let } D^{(k)}=(d^{(k)}{ij}) \text{ be a new } n \times n \text{ matrix}\ 6\ \t\t\bold{for\ } i = 1 \bold{\ to \ } n\ 7 \t\t\t \bold{for\ } j = 1\bold{\ to\ } n\ 8 \ \t\t\t\t d^{(k)}{ij}= \min(d^{k-1}{ij}, d^{k-1}{ik} + d^{k-1}_{kj})\ 9 \ \bold{return\ } D^{(n)}$
- This algorithm runs in
time.
1.2.1 - Floyd Warshall Algorithm Example
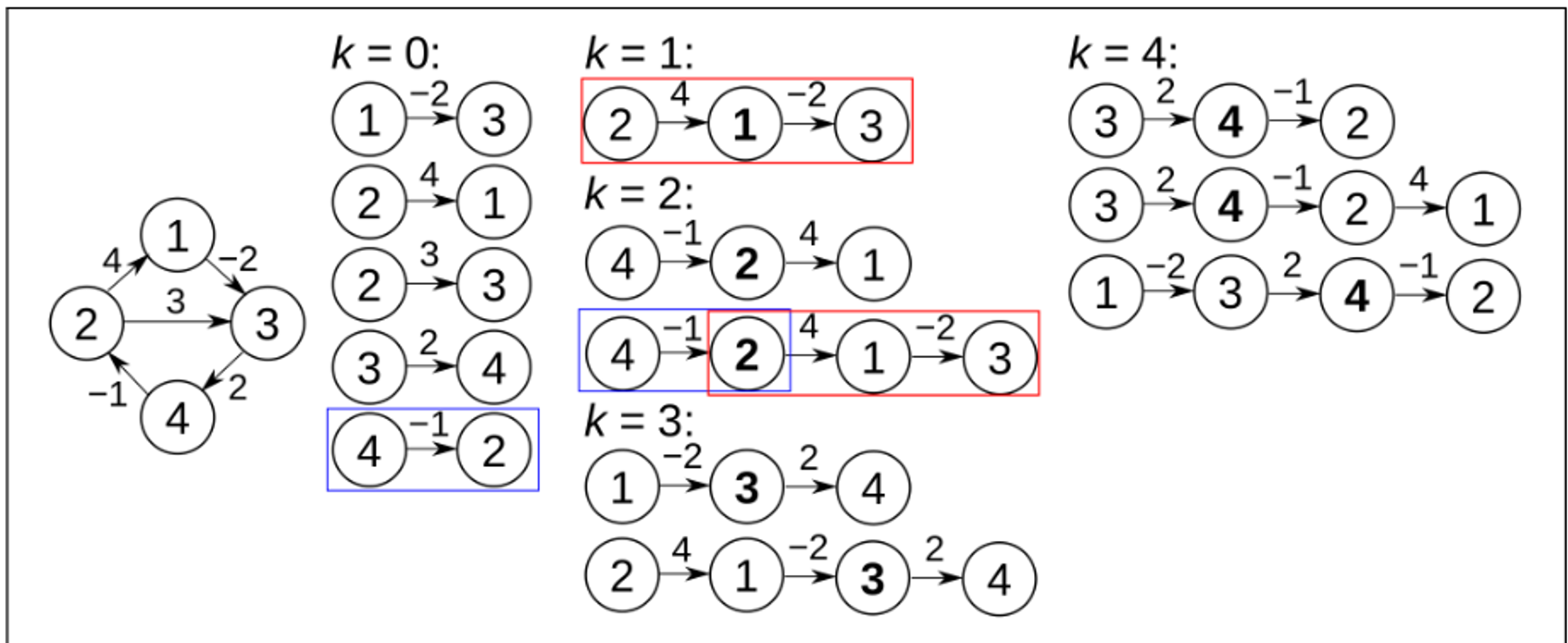
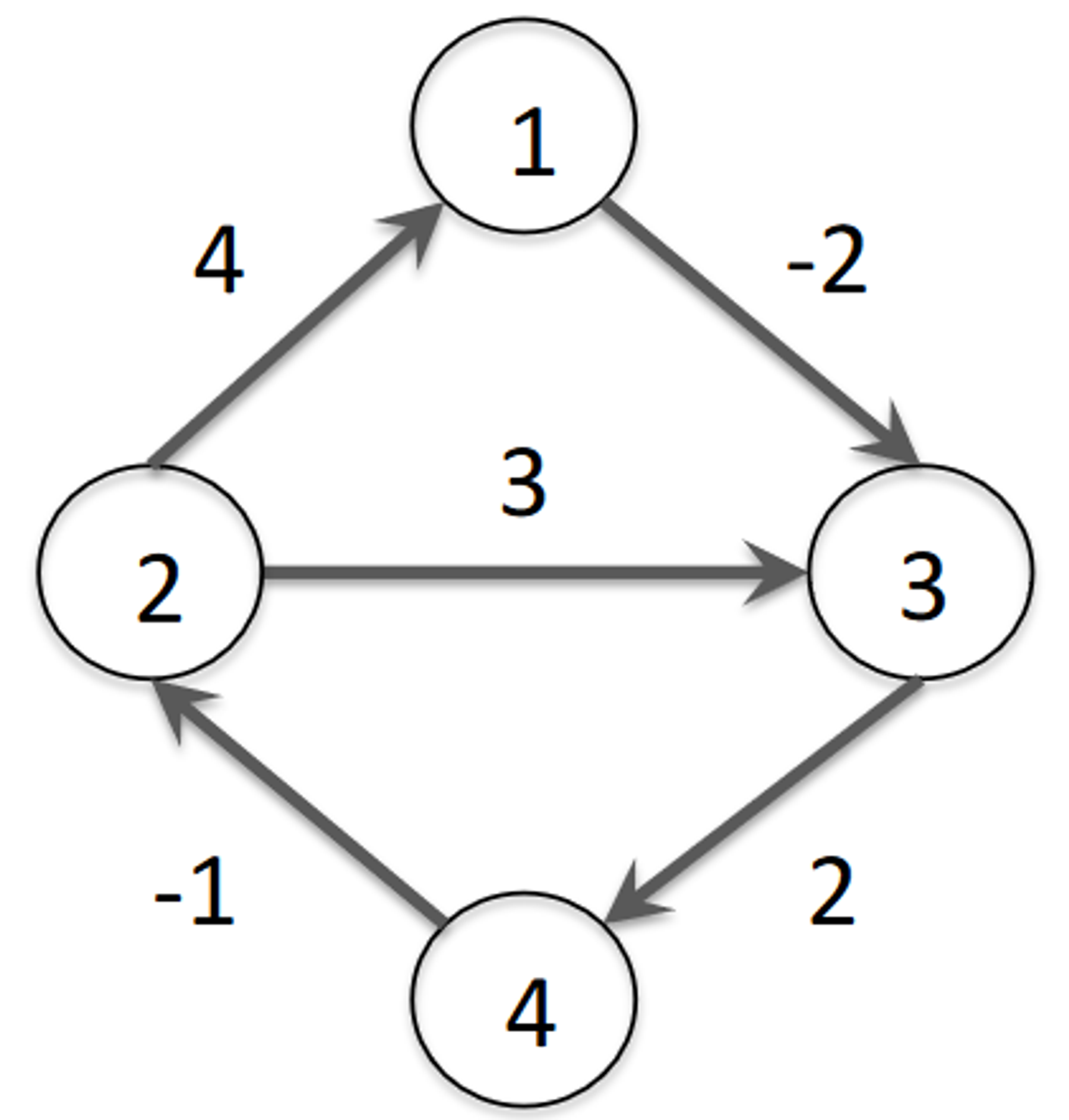
1.3 - Aside: Johnson’s Algorithm
- Johnson’s Algorithm is
in the worst case, but for sparse graphs is using an adjacency list representation - in practice this isn’t always faster as it has very large constants associated with it. - Our strategy is to
- Reweight to eliminate negative-weight edges
- Add a new source vertex.
- Run Dijkstra’s Algorithm for each node